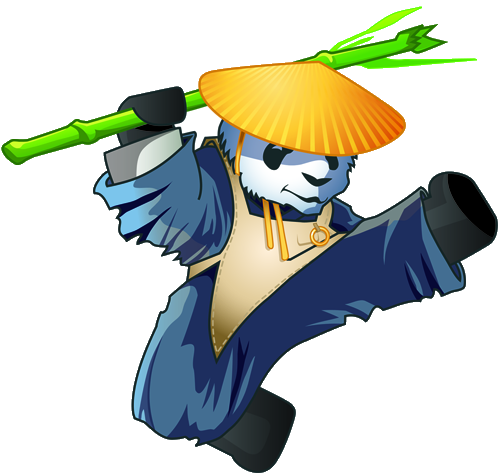
the stack
Base actions are push and pop, just like FILO(first in last out) queue.
base case and recursive case
Every recursive function has two parts: the base case, and the recursive case.
divide and conquer
Suppose you’re a farmer with a plot of land. You want to divide this farm evenly into square plots. You want the plots to be as big as possible. Just divide to get the largest square, and repeat the step with the remaining part.
inductive proofs
For example, suppose I want to prove that I can climb to the top of a ladder. In the inductive case, if my legs are on a rung, I can put my legs on the next rung. So if I’m on rung 2, I can climb to rung 3. That’s the inductive case.
Tower of Hanoi
The objective of the puzzle is to move the entire stack to another rod, obeying the following simple rules:
- Only one disk can be moved at a time.
- Each move consists of taking the upper disk from one of the stacks and placing it on top of another stack or on an empty rod.
- No disk may be placed on top of a smaller disk.
With 3 disks, the puzzle can be solved in 7 moves. The minimal number of moves required to solve a Tower of Hanoi puzzle is 2^n − 1, where n is the number of disks.
1 | #include <stdio.h> |
Fibonacci sequence
A typical recursive example, F(1)=1, F(2)=1, F(n)=F(n-1)+F(n-2) (n>=2,n∈N)
As described above, the base case is F(1)=1, F(2)=1, and the recursive case is F(n)=F(n-1)+F(n-2).
1 | #include <stdio.h> |
Some other fibonacci interesting sequences
- Fibonacci Brick Wall Patterns, The point of this activity is to see how many ways you can build a brick wall using the given amount of bricks.
- Each wall needs to be two units tall.
- Each brick standing on end is two units tall.
- Each brick laying on it’s side is one unit tall.
- You are first given one, then two, three, etc.
- For one brick there is only one method of building a wall. Two methods for two, and three methods for three.
- How many ways are there to build a wall with four bricks, five?Show your work, and how does this tie into the Fibonacci sequence?
quick sort
Another typical recursive example.
1 | #include <stdio.h> |
Pascal’s triangle
Reference
- 程序员的数学(日 结城浩)
- Algorithms, 4th Edition
- Grokking Algorithms: An illustrated guide for programmers and other curious people